Building a News Feed application with React Native
24 January 2023
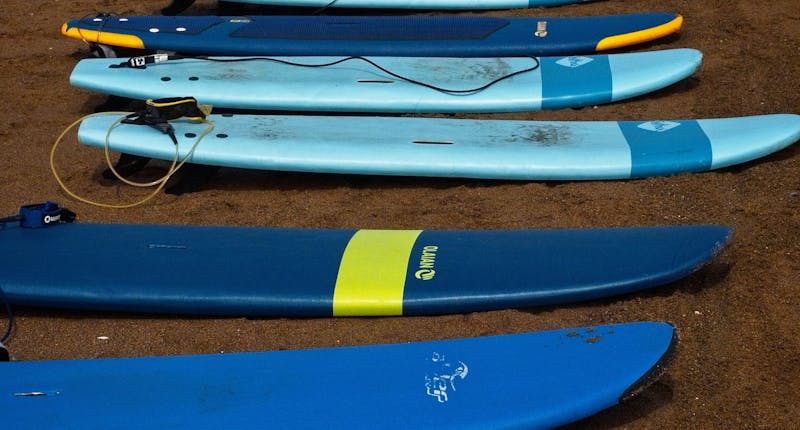
I am releasing a book about the React Native ecosystem, which covers everything I wish I had known before I started working with this technology.
If you appreciate my work and would like to show your support, please check the Road to React Native.
Yesterday I was speaking with Frank von Hoven about tooling for React Native. These days there are plenty of tools available like Amplify, Firebase, and Hasura to speed up the development of your mobile application.
Imagine you need to ship a social mobile application with a news feed like Twitter, Instagram, or Dribble. Under the hood you need to use WebSockets to have real-time updates, having a strong backend team of engineers, and spend months building the API.
Or you can use the stream API to ship your app with React Native in hours.
Introducing expo-activity-feed
When you are building a newsfeed, you need to use the prop refreshControl, the Linking component, and implement the logic yourself.
With expo-activity-feed, you can have an activity feed with pull to refresh and Linking in 5 minutes. It's super effective.
You need to wrap your application with StreamApp, add your API credentials, and add the FlatFeed.
import React from 'react';
import { SafeAreaProvider } from 'react-native-safe-area-context';
import SafeAreaView from 'react-native-safe-area-view';
import { StreamApp, FlatFeed } from 'expo-activity-feed';
function App() {
return (
<SafeAreaProvider>
<SafeAreaView style={{ flex: 1 }} forceInset={{ top: 'always' }}>
<StreamApp
apiKey={GETSTREAM_API_KEY}
appId={APP_ID}
token={GETSTREAM_API_TOKEN}
>
<FlatFeed feedGroup="timeline" userId="user-one" />
</StreamApp>
</SafeAreaView>
</SafeAreaProvider>
);
}
export default App;
And š„ your feed is ready.
The like button feature
You are happy but the marketing team needs a like button.
You can add a Footer render prop with LikeButton to your activity component.
import { LikeButton } from 'expo-activity-feed';
function CustomActivity(props) {
return <Activity {...props} Footer={
<LikeButton {...props} />
}
/>;
}
...
<FlatFeed
feedGroup="timeline"
userId="user-one"
Activity={CustomActivity}
/>
Add a status
If you want to add a publish a status feature, guess what? it's available on the stream API.
You need to use StatusUpdateForm with the same feedGroup name.
import { StatusUpdateForm } from 'expo-activity-feed';
<StatusUpdateForm feedGroup="timeline" />
Did you notice the problem here? Despite using SafeAreaView we have an issue with the keyboard.
It's normal. In the React Native world, you spend a ridiculous amount of time fixing bugs with the Keyboard on iOS AND Android.
I tested on the iPhone 12 Pro Max first, and I didn't encounter the issue with the iPhone SE.
ProTip: Always toggle the software keyboard when you are using a simulator to test behaviors.
Updating the feed
At this point, some backend work is involved. On the React Native code, you can just add the notify props to your FlatFeed
<FlatFeed
feedGroup="timeline"
userId="user-one"
Activity={CustomActivity}
notify
/>
Conclusion
If you want to quickly create a News Feed mobile application, Stream API is a great choice. At the time of writing this article, there were no TypeScript definitions, which was frustrating as I couldn't use the auto-import feature with the keyboard shortcut ā.
However, this is a minor detail compared to the time saved creating this small application with Expo and React Native. Finally, don't forget to use the correct Keyboard with your TextInput to have a delightful UX.
References
Hi, Iām David, a french freelance developer working remotely. Iām the author of this blog, nice to meet you!
Subscribe?
Be the first to receive insightful articles and actionable resources that help you to elevate your skills.