React Native Centering Made Simple: 11 Tips and Tricks
04 December 2022
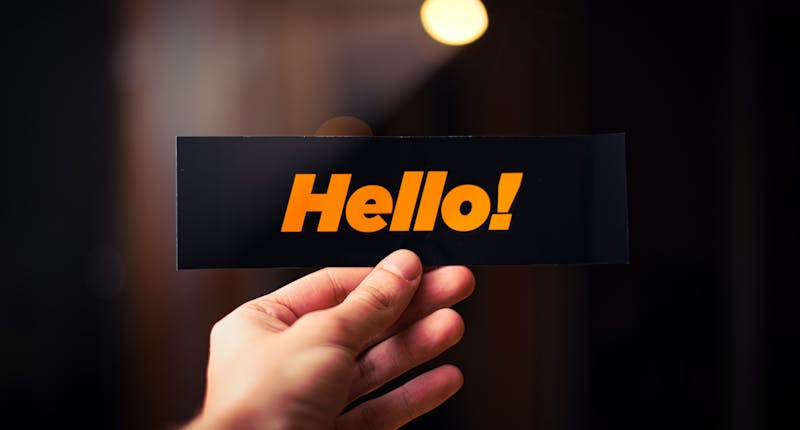
I am releasing a book about the React Native ecosystem, which covers everything I wish I had known before I started working with this technology.
If you appreciate my work and would like to show your support, please check the Road to React Native.
Centering views or text in React Native can sometimes be a challenging task, especially for beginners. In this blog post, we will provide 11 different ways to center views or text in React Native.
We will also provide code examples for each method so you can easily implement them in your own projects.
Use the flex and justifyContent styles to center views horizontally and vertically. For example:
<View style={{flex: 1, justifyContent: 'center', alignItems: 'center'}}>
<Text>Hello, World!</Text>
</View>
Use the alignSelf style on the child view to center it within its parent. For example:
<View style={{flex: 1}}>
<View style={{alignSelf: 'center'}}>
<Text>Hello, World!</Text>
</View>
</View>
Use the textAlign style to center text within a view. For example:
<View style={{flex: 1, justifyContent: 'center'}}>
<Text style={{textAlign: 'center'}}>Hello, World!</Text>
</View>
Use the position: 'absolute' and left, right, top, and bottom styles to position a view in the center of its parent. For example:
<View style={{flex: 1, position: 'relative'}}>
<View style={{position: 'absolute', left: 0, right: 0, top: 0, bottom: 0}}>
<Text>Hello, World!</Text>
</View>
</View>
Use the position: 'relative' and margin: 'auto' styles to center a view within its parent. For example:
<View style={{flex: 1, position: 'relative'}}>
<View style={{position: 'relative', margin: 'auto'}}>
<Text>Hello, World!</Text>
</View>
</View>
Use the transform: [{translateX: '-50%'}, {translateY: '-50%'}] styles to center a view within its parent. For example:
<View style={{flex: 1, position: 'relative'}}>
<View style={{position: 'absolute', left: 0, right: 0, top: 0, bottom: 0, transform: [{translateX: '-50%'}, {translateY: '-50%'}]}}>
<Text>Hello, World!</Text>
</View>
</View>
Use the Dimensions API to get the width and height of the screen, and then use the left and top styles to position the view in the center of the screen. For example:
import {Dimensions} from 'react-native';
const screenWidth = Dimensions.get('window').width;
const screenHeight = Dimensions.get('window').height;
<View style={{flex: 1, position: 'absolute', left: screenWidth / 2, top: screenHeight / 2}}>
How do you center a div in the middle of the page vertically?
To center a view in the middle of the screen vertically in React Native, you can use the flex and justifyContent styles. For example:
<View style={{flex: 1, justifyContent: 'center'}}>
<View>
{/* View content goes here */}
</View>
</View>
This will cause the child view to be vertically centered within its parent view. You can also use the alignItems style to center the view horizontally within its parent view. For example:
<View style={{flex: 1, justifyContent: 'center', alignItems: 'center'}}>
<View>
{/* View content goes here */}
</View>
</View>
You can also use the alignSelf style on the child view to center it within its parent. For example:
<View style={{flex: 1}}>
<View style={{alignSelf: 'center'}}>
{/* View content goes here */}
</View>
</View>
These methods will allow you to easily center a view in the middle of the screen vertically in React Native.
Centering lines of text
To center lines of text in React Native, you can use the textAlign style. For example:
<View>
<Text style={{textAlign: 'center'}}>
This text will be centered
</Text>
</View>
This will center the lines of text within the parent view. You can also use the justifyContent and alignItems styles on the parent view to center the text both horizontally and vertically within the view. For example:
<View style={{justifyContent: 'center', alignItems: 'center'}}>
<Text>
This text will be centered both horizontally and vertically
</Text>
</View>
These examples demonstrate different methods for centering things in React Native. You can use these methods to center views, text, or other elements within their parent containers.
Hi, I’m David, a french freelance developer working remotely. I’m the author of this blog, nice to meet you!
Subscribe?
Be the first to receive insightful articles and actionable resources that help you to elevate your skills.