Get an overview of all keyboard types available in React Native for both iOS and Android
03 May 2023
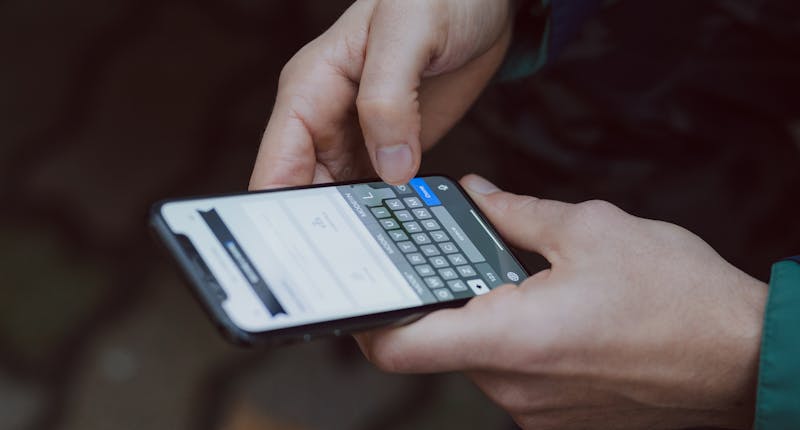
I am releasing a book about the React Native ecosystem, which covers everything I wish I had known before I started working with this technology.
If you appreciate my work and would like to show your support, please check the Road to React Native.
During your life as a React Native developer, you are going to handle a lot of different scenarios using keyboards. You need to know all possible combinations to choose the one that corresponds to your situation.
In this article, we are going to define and compare all types of keyboards available in react-native. We will discuss how to create a numeric keyboard that includes a decimal point for iOS and Android devices.
This can be useful for applications that require users to input numerical data with decimal points, such as financial or scientific calculations. We will also discuss creating a numeric keypad only for iOS devices and a keyboard layout with numbers only for iPhone devices. Additionally, we will explore how to prevent users from entering non-numeric characters and how to add a "search" button to clear the input field. If you want to learn more about customizing keyboard layouts in React Native, please continue reading or feel free to contact me for more information.
The problem
Imagine it's Monday morning, you have a new bubble on slack, your lovely designer wants small feedback for his call to action...
It's a good practice for mobile Call To Action to be within the thumb reach.
💯 Indeed, but from a technical perspective, the React Native platform comes with some limits.
How to use the Input Keyboard type in React Native
This is an example of a controlled component in React Native.
import { TextInput } from "react-native";
const App = () => {
const [inputValue, setInputValue] = useState("Example text");
return (
<View>
<TextInput
onChangeText={setInputValue}
value={inputValue}
/>
</View>
);
};
TextInput props values for `keyboardType` by example
- default
- numeric
- password
- email-address
- phone-pad
- ascii-capable
- numbers-and-punctuation
- url
- number-pad
- name-phone-pad
- decimal-pad
- web-search
Protip to toggle the iOS keyboard on macOS
If you are using the macOS simulator to work on iOS mobile, you will need to toggle the keyboard or it will never appear (IO > Keyboard > Toggle Software Keyboard)
All React Native keyboardType examples (iOS on the left, Android on the right)
With all the screenshots above, we are using this code and changing only keyboardType props. The source code is available on GitHub.
<TextInput
onChangeText={setInputValue}
value={inputValue}
keyboardType="numeric" <---- the props we are changing
/>
`default`
This is the basic keyboard when you just want to handle data from a TextInput.
`numeric`
Numeric keyboards are used when you need to take data as a number. As you can see the Android keyboard is a little bit different, the user can have access to string characters on his screen while apple doesn't show them.
`password`
When the user wants to write his password, the first letter is not always a capital letter that's why we need to use this type of keyboard for password input.
`email-address`
For the Email type, the keyboard is basic and we can easily have access to the "@" and the "." character.
As you can see the type defines the phone suggestion and I can select faster my mail address.
Phone-pad type
The phone-pad type is the keyboard that we use when we made a phone call. We have access to special characters like #,+,*.
Even if on Android the keyboard is a little bit different, this is the principal functionality of this keyboard.
`ascii-capable`
`numbers-and-punctuation`
`url`
When the user wants to find a website, he needs to have ".", "/" and the domain extension quickly
`number-pad`
When you are asking for a birthdate for example.
`name-phone-pad`
`decimal`
`twitter`
`web-search`
Best way to add a "Done" button in React Native
People are struggling to quickly send information, which can cause the same problem as before. Remember, you're on a mobile device—every millisecond counts!
Many React developers are asking me: what is the best way to add a "Done" button with a React Native keyboard? Creating your own button component takes time and won't have the platform's look and feel. To quickly attach a keyboard event, you can use the returnKeyType prop.
TextInput props values for `returnKeyType` by example
- default
- go
- join
- next
- route
- search
- send
- yahoo
- done
- emergency-call
<TextInput
onChangeText={setInputValue}
value={inputValue}
returnKeyType="done" <---- the props we are changing
/>
Here is a preview in gif with all the variations on iOS.
How to avoid default keyboard word suggestions?
Depending on your Android keyboard settings, you can have various default words or suggestions when you fill in a TextInput. Android offers more options than iOS, even with the availability of custom keyboard apps such as Grammarly nowadays.
Word suggestion can be a great feature when messaging or filling out a description, but sometimes it can be a nightmare, adding extra noise for no reason. We need a way to control its behavior, such as being disabled when requiring an email.
<TextInput
onChangeText={setInputValue}
value={inputValue}
placeholder="hello@g2i.com"
keyboardType="email-address" // to add @ on the keyboard
autoCapitalize="none" // no uppercase please
autoComplete="email" // for android
autoCorrect={false} // to disable suggested words
/>
Here is a preview with iOS, we have disabled suggested words and we autocomplete email, this UX sparks joy!
This article does not cover many other topics, such as enabling iOS to autofill a TextInput for incoming SMS messages. If you notice any other missing information, please let me know on Twitter and I will publish an update.
GitHub Code
Hi, I’m David, a french freelance developer working remotely. I’m the author of this blog, nice to meet you!
Subscribe?
Be the first to receive insightful articles and actionable resources that help you to elevate your skills.